Loading & Training Models on Kaggle Datasets: A Complete Guide
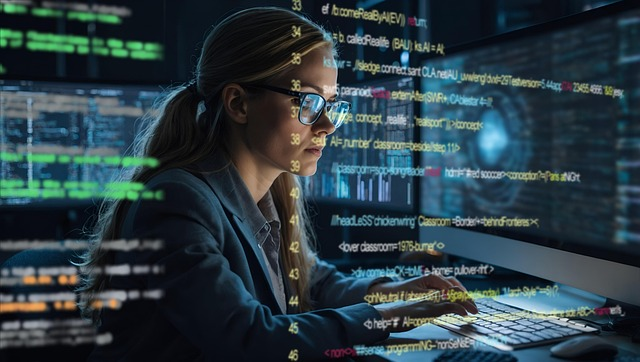
Introduction: Unlock the Power of Kaggle for Your Machine Learning Projects
Have you been struggling to find quality datasets for your machine learning projects? Or perhaps you’ve found great datasets but aren’t sure how to efficiently load and use them in your models? In this comprehensive guide, we’ll explore how to leverage Kaggle’s vast repository of datasets and powerful computing environment to build production-ready machine learning models.
Kaggle has revolutionized the data science landscape by providing free access to thousands of datasets, computing resources, and a platform for collaboration. Whether you’re working with structured CSV data or complex image datasets, this tutorial will walk you through the entire process from data access to model deployment.
What Makes Kaggle an Essential Tool
Kaggle offers several advantages that make it indispensable for data scientists and machine learning practitioners:
- Massive Dataset Repository: Access to thousands of free, high-quality datasets across various domains
- Free Computational Resources: GPU and TPU access without any setup hassles
- Collaborative Environment: Learn from and build upon others’ work
- Competitive Platform: Sharpen your skills through competitions
- Version Control: Keep track of your notebook iterations
- Community Support: Get help from millions of data enthusiasts
By the end of this tutorial, you’ll be able to harness these benefits to accelerate your machine learning projects.
Setting Up Your Kaggle Environment
Before diving into datasets, let’s ensure your Kaggle environment is properly configured:
1. Create and Set Up Your Account
If you haven’t already, sign up for a Kaggle account. Verify your phone number to unlock full capabilities, including GPU access.
2. Set Up Your Profile
Complete your profile to build credibility within the community. This increases the visibility of your notebooks and datasets.
3. Understand Kaggle Notebooks (Kernels)
Kaggle Notebooks are interactive coding environments that run in the cloud. They come with:
- Pre-installed data science libraries
- Option to use CPU, GPU, or TPU
- Up to 9 hours of continuous runtime
- 20GB of disk space
4. Configure Your Notebook Settings
When creating a new notebook, click on the “…” menu and select “Settings” to configure:
- Hardware accelerator (None/GPU/TPU)
- Internet access (on/off)
- Dataset connections
# Check available resources in your Kaggle notebook
import os
import psutil
import tensorflow as tf
import torch
# CPU info
print(f"Number of CPU cores: {os.cpu_count()}")
print(f"Available memory: {psutil.virtual_memory().available / (1024 ** 3):.2f} GB")
# GPU info (if available)
try:
print(f"TensorFlow GPU available: {tf.config.list_physical_devices('GPU')}")
print(f"PyTorch GPU available: {torch.cuda.is_available()}")
if torch.cuda.is_available():
print(f"GPU Model: {torch.cuda.get_device_name(0)}")
except:
print("GPU check failed - libraries might not be installed")
Working with CSV Datasets
Finding and Adding Datasets
- Browse to Kaggle Datasets
- Search for relevant datasets using keywords
- Once you find a dataset, click “Add” to use it in your notebook
Loading CSV Data
When working with structured data in CSV format, pandas is your best friend:
# Import necessary libraries
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
# Option 1: Load dataset that you've added to your notebook
df = pd.read_csv('../input/dataset-name/file.csv')
# Option 2: Load directly using Kaggle's API
# First, find the dataset API command from the dataset page
!kaggle datasets download -d username/dataset-name
!unzip dataset-name.zip
df = pd.read_csv('file.csv')
# Basic exploration
print(f"Dataset shape: {df.shape}")
print("\nFirst 5 rows:")
print(df.head())
print("\nData types:")
print(df.dtypes)
print("\nMissing values:")
print(df.isnull().sum())
Data Preprocessing for CSV Data
# Handle missing values
df = df.fillna(df.mean()) # Fill numeric columns with mean
# OR
df = df.dropna() # Remove rows with missing values
# Encode categorical variables
from sklearn.preprocessing import LabelEncoder, OneHotEncoder
le = LabelEncoder()
df['category_encoded'] = le.fit_transform(df['category'])
# Alternative: One-hot encoding
df_encoded = pd.get_dummies(df, columns=['category'])
# Feature scaling
from sklearn.preprocessing import StandardScaler, MinMaxScaler
scaler = StandardScaler()
df[['feature1', 'feature2']] = scaler.fit_transform(df[['feature1', 'feature2']])
# Split data
from sklearn.model_selection import train_test_split
X = df.drop('target', axis=1)
y = df['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Working with Image Datasets
Kaggle is particularly powerful for computer vision projects due to its GPU capabilities and vast image datasets.
Finding and Adding Image Datasets
The process is similar to CSV datasets, but pay attention to:
- Total size (ensure it fits within Kaggle’s limits)
- Structure (folder organization)
- Format (JPEG, PNG, etc.)
Loading and Preprocessing Images
# Import necessary libraries
import os
import cv2
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
from glob import glob
# Option 1: Load images using PIL
image_dir = '../input/dataset-name/images/'
image_paths = glob(os.path.join(image_dir, '*.jpg'))
# Display a sample image
img = Image.open(image_paths[0])
plt.figure(figsize=(8, 8))
plt.imshow(img)
plt.axis('off')
plt.show()
print(f"Total images found: {len(image_paths)}")
Creating Image Datasets for Deep Learning
With TensorFlow/Keras:
import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Set parameters
img_height, img_width = 224, 224
batch_size = 32
# Create data generators with augmentation
train_datagen = ImageDataGenerator(
rescale=1./255,
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
validation_split=0.2
)
# Flow from directory
train_generator = train_datagen.flow_from_directory(
'../input/dataset-name/train',
target_size=(img_height, img_width),
batch_size=batch_size,
class_mode='categorical',
subset='training'
)
validation_generator = train_datagen.flow_from_directory(
'../input/dataset-name/train',
target_size=(img_height, img_width),
batch_size=batch_size,
class_mode='categorical',
subset='validation'
)
# Display class names
class_names = list(train_generator.class_indices.keys())
print(f"Classes: {class_names}")
With PyTorch:
from torch.utils.data import Dataset, DataLoader
from torchvision import transforms
import torch
# Custom dataset class
class ImageDataset(Dataset):
def __init__(self, image_paths, labels=None, transform=None):
self.image_paths = image_paths
self.labels = labels
self.transform = transform
def __len__(self):
return len(self.image_paths)
def __getitem__(self, idx):
img_path = self.image_paths[idx]
image = Image.open(img_path).convert('RGB')
if self.transform:
image = self.transform(image)
if self.labels is not None:
return image, self.labels[idx]
else:
return image
# Create transforms
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# Create dataset and dataloader
dataset = ImageDataset(image_paths, labels, transform=transform)
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
Training Machine Learning Models
Now that we have our data ready, let’s train some models. We’ll cover both traditional ML and deep learning approaches.
Traditional ML with Scikit-learn (for CSV data)
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report, confusion_matrix
# Train a Random Forest model
rf_model = RandomForestClassifier(n_estimators=100, random_state=42)
rf_model.fit(X_train, y_train)
# Make predictions
y_pred = rf_model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.4f}")
print("\nClassification Report:")
print(classification_report(y_test, y_pred))
# Plot confusion matrix
plt.figure(figsize=(8, 6))
sns.heatmap(confusion_matrix(y_test, y_pred), annot=True, fmt='d', cmap='Blues')
plt.xlabel('Predicted')
plt.ylabel('Actual')
plt.title('Confusion Matrix')
plt.show()
# Feature importance
feature_importance = pd.DataFrame({
'Feature': X_train.columns,
'Importance': rf_model.feature_importances_
}).sort_values('Importance', ascending=False)
plt.figure(figsize=(10, 6))
sns.barplot(x='Importance', y='Feature', data=feature_importance.head(10))
plt.title('Top 10 Important Features')
plt.tight_layout()
plt.show()
Deep Learning with TensorFlow/Keras (for Image data)
from tensorflow.keras.applications import MobileNetV2
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D, Dropout
from tensorflow.keras.optimizers import Adam
# Build the model using transfer learning
base_model = MobileNetV2(weights='imagenet', include_top=False, input_shape=(img_height, img_width, 3))
base_model.trainable = False # Freeze the base model
model = Sequential([
base_model,
GlobalAveragePooling2D(),
Dense(1024, activation='relu'),
Dropout(0.2),
Dense(len(class_names), activation='softmax')
])
# Compile the model
model.compile(
optimizer=Adam(learning_rate=0.001),
loss='categorical_crossentropy',
metrics=['accuracy']
)
# Model summary
model.summary()
# Train the model
history = model.fit(
train_generator,
validation_data=validation_generator,
epochs=10,
callbacks=[
tf.keras.callbacks.EarlyStopping(patience=3, restore_best_weights=True),
tf.keras.callbacks.ReduceLROnPlateau(factor=0.1, patience=2)
]
)
# Plot training history
plt.figure(figsize=(12, 4))
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='Train')
plt.plot(history.history['val_accuracy'], label='Validation')
plt.title('Accuracy')
plt.legend()
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='Train')
plt.plot(history.history['val_loss'], label='Validation')
plt.title('Loss')
plt.legend()
plt.tight_layout()
plt.show()
Deep Learning with PyTorch (for Image data)
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import models
from tqdm.notebook import tqdm
# Check if GPU is available
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Using device: {device}")
# Create the model using transfer learning
model = models.resnet18(pretrained=True)
num_features = model.fc.in_features
model.fc = nn.Linear(num_features, len(class_names))
model = model.to(device)
# Loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
scheduler = optim.lr_scheduler.ReduceLROnPlateau(optimizer, mode='min', patience=1, factor=0.5)
# Training loop
num_epochs = 10
train_losses = []
val_losses = []
train_accuracies = []
val_accuracies = []
for epoch in range(num_epochs):
# Training
model.train()
running_loss = 0.0
correct = 0
total = 0
for inputs, labels in tqdm(train_dataloader, desc=f"Epoch {epoch+1}/{num_epochs} [Train]"):
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item() * inputs.size(0)
_, predicted = torch.max(outputs, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
epoch_loss = running_loss / len(train_dataset)
epoch_acc = correct / total
train_losses.append(epoch_loss)
train_accuracies.append(epoch_acc)
# Validation
model.eval()
running_loss = 0.0
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in tqdm(val_dataloader, desc=f"Epoch {epoch+1}/{num_epochs} [Val]"):
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
loss = criterion(outputs, labels)
running_loss += loss.item() * inputs.size(0)
_, predicted = torch.max(outputs, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
epoch_loss = running_loss / len(val_dataset)
epoch_acc = correct / total
val_losses.append(epoch_loss)
val_accuracies.append(epoch_acc)
scheduler.step(epoch_loss)
print(f"Epoch {epoch+1}/{num_epochs} - "
f"Train Loss: {train_losses[-1]:.4f}, Train Acc: {train_accuracies[-1]:.4f}, "
f"Val Loss: {val_losses[-1]:.4f}, Val Acc: {val_accuracies[-1]:.4f}")
Optimizing Performance
To get the most out of Kaggle’s resources and improve your model performance:
Memory Efficiency Tips
# 1. Use generators instead of loading everything into memory
# 2. Delete unnecessary variables
del large_variable
import gc
gc.collect()
# 3. Process data in chunks
for chunk in pd.read_csv('../input/large-dataset.csv', chunksize=10000):
# Process each chunk
process_data(chunk)
Speed Optimizations
# 1. Use GPU when available for deep learning tasks
# 2. Enable mixed precision training in TensorFlow
from tensorflow.keras.mixed_precision import experimental as mixed_precision
policy = mixed_precision.Policy('mixed_float16')
mixed_precision.set_global_policy(policy)
# 3. For PyTorch, use AMP (Automatic Mixed Precision)
from torch.cuda.amp import autocast, GradScaler
scaler = GradScaler()
# Inside training loop
with autocast():
outputs = model(inputs)
loss = criterion(outputs, labels)
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
Saving and Sharing Your Work
One of Kaggle’s strengths is the ability to save and share your work with the community:
Saving Models
# TensorFlow/Keras
model.save('model.h5')
# PyTorch
torch.save(model.state_dict(), 'model.pth')
# Scikit-learn
import joblib
joblib.dump(rf_model, 'random_forest_model.joblib')
Creating Output Files
# For kaggle competitions - create submission file
submission = pd.DataFrame({
'id': test_df['id'],
'prediction': y_pred
})
submission.to_csv('submission.csv', index=False)
# Download files from Kaggle notebook
from IPython.display import FileLink
FileLink('model.h5') # Creates download link in the notebook
Version Control and Sharing
- Click “Save Version” to create a snapshot of your notebook
- Add a version name and description
- Choose visibility (Public/Private)
- Click “Save” to commit your changes
- Share using the “Share” button to get a public URL
Common Challenges and Solutions
1. Dataset Size Limitations
Challenge: Kaggle has a 20GB disk space limit.
Solution:
- Use data generators to process data in batches
- Focus on a subset of the dataset for development
- Use efficient data formats like parquet instead of CSV
2. Session Timeouts
Challenge: Kaggle notebooks time out after 9 hours.
Solution:
- Save checkpoints frequently
- Design workflows that can resume from checkpoints
- Break large tasks into smaller notebooks
# Save checkpoint in TensorFlow/Keras
checkpoint_path = "training_checkpoint.ckpt"
checkpoint_callback = tf.keras.callbacks.ModelCheckpoint(
filepath=checkpoint_path,
save_weights_only=True,
save_best_only=True
)
model.fit(train_data, epochs=50, callbacks=[checkpoint_callback])
# Load checkpoint
model.load_weights(checkpoint_path)
3. Package Version Issues
Challenge: Kaggle may not have the exact version of a package you need.
Solution:
- Use
!pip install
to install specific versions - Check Kaggle’s package list before planning your approach
- Design your code to be version-flexible
# Install specific versions
!pip install -q transformers==4.26.0
Next Steps
Now that you’ve learned how to load, process, and train models on Kaggle datasets, here are some ways to build on your skills:
- Join a Kaggle Competition: Apply your knowledge in a competitive setting
- Create and Share Datasets: Contribute to the community by uploading your own curated datasets
- Explore Advanced Techniques: Try ensemble methods, hyperparameter tuning, or more complex architectures
- Collaborate with Others: Fork and improve on popular notebooks
- Deploy Your Models: Learn how to take models from Kaggle to production environments
Final Tips for Success
- Start Small: Begin with manageable datasets before tackling larger ones
- Learn from Others: Study top-rated notebooks in your area of interest
- Document Well: Add markdown cells explaining your approach
- Optimize Iteratively: First make it work, then make it fast
- Share Your Insights: Engage with the community through discussions
Conclusion
Kaggle provides everything you need to go from data to trained model in one platform. By mastering the techniques covered in this guide, you’ve gained the essential skills to leverage Kaggle’s resources effectively for your machine learning projects.
Remember that the best way to learn is by doing. Start applying these techniques to real datasets today, and join the millions of data scientists using Kaggle to develop and showcase their skills.